How to setup Posthog on Next.js
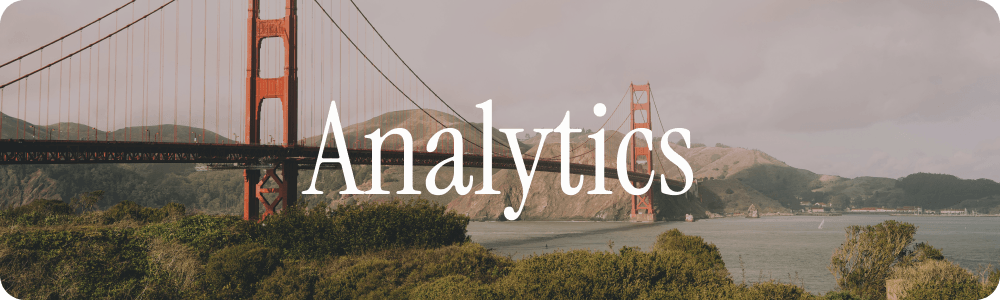
Recently, I started collecting high-level analytics for my website. I use PostHog because of its generous free tier and powerful features. While it’s a great service, I ran into a few points of confusion during setup. To save you the same trouble, here’s my step-by-step guide to integrating PostHog with Next.js w/ a reverse proxy.
Prerequisites
- Posthog cloud or selfhosted
- Next.js
Client Setup
Since PostHog doesn’t provide framework-specific libraries, we’ll use Posthog-js for our integration.
# Install PostHog
yarn add posthog-js
npm install --save posthog-js
pnpm add posthog-js
Next, you’ll need your Project API key, which you can find in your PostHog portal. We’ll store this in environment variables:
- POSTHOG_API_KEY → Your project’s API key
- POSTHOG_URL → The PostHog instance URL (check whether you’re on the US or EU domain)
// .env
NEXT_PUBLIC_POSTHOG_KEY=posthog api key
NEXT_PUBLIC_POSTHOG_HOST=https://us.i.posthog.com
Providers
To track analytics across our app, we need to wrap it in a provider. Instead of relying on PostHog’s built-in tracking, we’ll manually capture page views, since Next.js doesn’t fire navigation events by default.
// app/providers.tsx
"use client";
import posthog from "posthog-js";
import { PostHogProvider as PHProvider } from "posthog-js/react";
import { useEffect } from "react";
export function PostHogProvider({ children }: { children: React.ReactNode }) {
useEffect(() => {
posthog.init(process.env.NEXT_PUBLIC_POSTHOG_KEY as string, {
api_host: process.env.NEXT_PUBLIC_POSTHOG_HOST as string,
capture_pageview: false,
capture_pageleave: true,
});
}, []);
return <PHProvider client={posthog}>{children}</PHProvider>;
}
We use "use client";
to ensure PostHog doesn’t load on the server. This doesn’t make your entire app client-side — your static and SSR routes will still work as expected. You can verify this in Next.js by checking the route status.
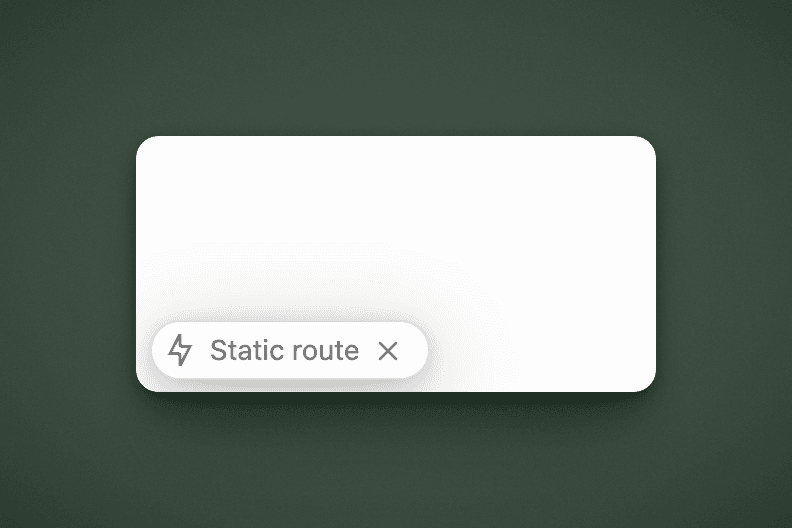
Manual Page View Tracking
Since we’ve disabled PostHog’s default page view tracking, we need to implement our own.
// app/PostHogPageView.tsx
"use client";
import { usePathname, useSearchParams } from "next/navigation";
import { useEffect, Suspense } from "react";
import { usePostHog } from "posthog-js/react";
function PostHogPageView(): null {
const pathname = usePathname();
const searchParams = useSearchParams();
const posthog = usePostHog();
useEffect(() => {
if (pathname && posthog) {
let url = window.origin + pathname;
if (searchParams.toString()) {
url = url + `?${searchParams.toString()}`;
}
posthog.capture("$pageview", { $current_url: url });
}
}, [pathname, searchParams, posthog]);
return null;
}
export default function SuspendedPostHogPageView() {
return (
<Suspense fallback={null}>
<PostHogPageView />
</Suspense>
);
}
Since PostHogPageView needs to access Next.js navigation events, we wrap it in a Suspense boundary to prevent hydration errors.
Next, we update our provider to include it:
// app/providers.tsx
"use client";
import posthog from "posthog-js";
import { PostHogProvider as PHProvider } from "posthog-js/react";
import { useEffect } from "react";
import PostHogPageView from "./PostHogPageView";
export function PostHogProvider({ children }: { children: React.ReactNode }) {
useEffect(() => {
posthog.init(process.env.NEXT_PUBLIC_POSTHOG_KEY as string, {
api_host: process.env.NEXT_PUBLIC_POSTHOG_HOST as string,
capture_pageview: false,
capture_pageleave: true,
});
}, []);
return (
<PHProvider client={posthog}>
<PostHogPageView />
{children}
</PHProvider>
);
}
Next.js < 15
If you’re using Next.js < 15, you might run into issues with <Suspense>
rendering on the server. In another project, I encountered this problem, and the fix was to dynamically import PostHogPageView:
// app/providers.tsx
import dynamic from "next/dynamic";
const PostHogPageView = dynamic(() => import("./PostHogPageView"), {
ssr: false,
});
Applying the Provider
Now, let’s add the PostHogProvider to our global layout.
// app/layout.tsx
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<PostHogProvider>
<body>{children}</body>
</PostHogProvider>
</html>
);
}
Make sure it’s inside the <html>
tag — not above it. I spent 30 minutes confused because I put the provider in the wrong place.
That’s it! If you’re not using a reverse proxy, you can stop here.
Reverse Proxy
By default, us.posthog.com
is blocked by most ad blockers and privacy tools. While I strongly support user privacy, this unfortunately prevents analytics from working. A reverse proxy helps solve this by routing requests through your own domain instead of PostHog’s, reducing blocking issues.
For whatever reason, the official PostHog guides didn’t work for me, despite extensive tinkering with Vercel rewrites and middleware. Vercel rewrites are ideal, but due to Vercel’s rewrite system, they consistently throw CORS errors.
My solution is to use Next.js rewrites directly. First, we’ll add the necessary redirects to the config.
- If you have existing rewrites, make sure they don’t override the new ones. For example, a rewrite like
source: "/*"
will take precedence over everything else.
// next.config.ts
import { type NextConfig } from "next";
const nextConfig: NextConfig = {
async rewrites() {
return [
{
source: "/ingest/static/:path*",
destination: "https://us-assets.i.posthog.com/static/:path*",
},
{
source: "/ingest/:path*",
destination: "https://us.i.posthog.com/:path*",
},
{
source: "/ingest/decide",
destination: "https://us.i.posthog.com/decide",
},
];
},
skipTrailingSlashRedirect: true,
};
export default nextConfig;
Now, we update our provider to point to the new rewrite destinations.
// app/PostHogPageView.tsx
"use client";
import posthog from "posthog-js";
import { PostHogProvider as PHProvider } from "posthog-js/react";
import { useEffect } from "react";
import PostHogPageView from "./PostHogPageView";
export function PostHogProvider({ children }: { children: React.ReactNode }) {
useEffect(() => {
posthog.init(process.env.NEXT_PUBLIC_POSTHOG_KEY as string, {
api_host: "/ingest",
ui_host: "https://us.posthog.com",
capture_pageview: false,
capture_pageleave: true,
});
}, []);
return (
<PHProvider client={posthog}>
<PostHogPageView />
{children}
</PHProvider>
);
}
Remember to add ui_host to ensure that PostHog still recognizes your original domain, preventing any issues in tracking.
That’s it
That’s everything you need to get PostHog working with a reverse proxy. If everything is set up correctly, you should start seeing analytics data appear in your PostHog portal.
"Data beats opinions.” - Marissa Mayer